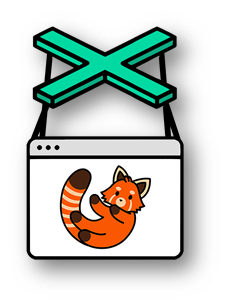
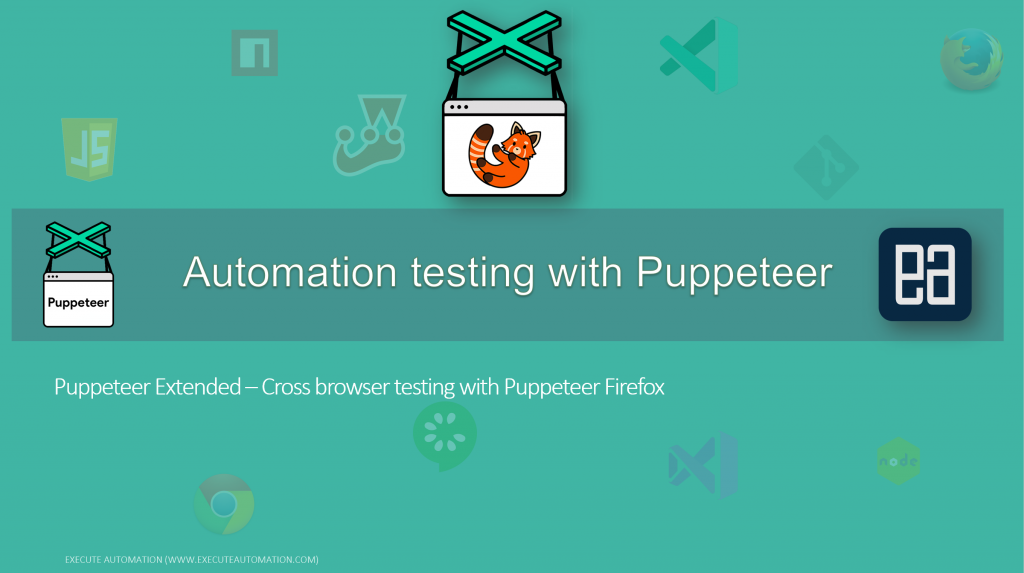
In this article, we will discuss cross-browser testing with Firefox and Chrome in Puppeteer
Puppeteer is a Node library which provides a high-level API to control Chrome or Chromium over the DevTools Protocol. Puppeteer runs headless by default, but can be configured to run full (non-headless) Chrome or Chromium.
Puppeteer Firefox
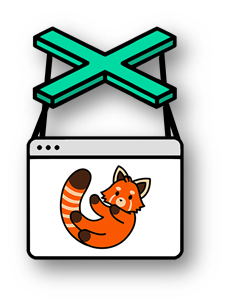
Puppeteer Firefox library is an additional library which is used to run puppeteer code on firefox browser. Basically Puppeteer firefox will download a custom-built Firefox (Firefox/63.0.4) that are guaranteed to work with the API.
Installing Puppeteer Firefox
Installing Puppeteer firefox is as simple as installing an npm package in your existing code, just type the below command to install puppeteer firefox in your existing project
Writing code to run on both Firefox and Chrome browser
const test = async browser => { //Page const page = await browser.newPage(); //Set view port of the page await page.setViewport({ "width": 1440, "height": 10000 }); await page.goto('http://executeautomation.com/demosite/Login.html'); await page.type('[name=UserName]', 'executeautomation'); await page.type('[name=Password]', 'admin'); await page.keyboard.press('Enter', { delay: 2000 }); //hover await page.hover("[id='Automation Tools']"); await browser.close(); }
The above code actually is the actual test code, but as you can see we are passing the browser as the parameter for the method to execute. This helps us to pass chrome or firefox browser to execute the above code. This can be achieved with the below code
const chrome = await puppeteerChrome.launch({ "headless": false, "slowMo": 50 }); //Chrome browser await test(chrome); const firefox = await puppeteerFirefox.launch({ "headless": false, "slowMo": 50 }); //firefox browser await test(firefox);
Here is the complete video of the above code and article
The above video is part of the Udemy course on Puppeteer available here https://www.udemy.com/course/puppeteer/
Please let me know if you are interested in the course, I will share you the coupon code for discount which you can use while purchasing the course.
Kindly share Discount coupon to buy this course
Please check your inbox for discount coupon code
Thanks
Kindly Share coupon code to buy this course.
Please check your inbox for discount coupon code
Thanks
Hi,
i Would lik a promo code. Many thanks in advance
Can you please send me another valid email address ?
Can you please share coupon code for Udemy Puppeteer curse
Please check your inbox for discount coupon code
Thanks
Hi,
Could I please get the coupon code for your course
Thank you
Please check your inbox for discount coupon code
Thanks