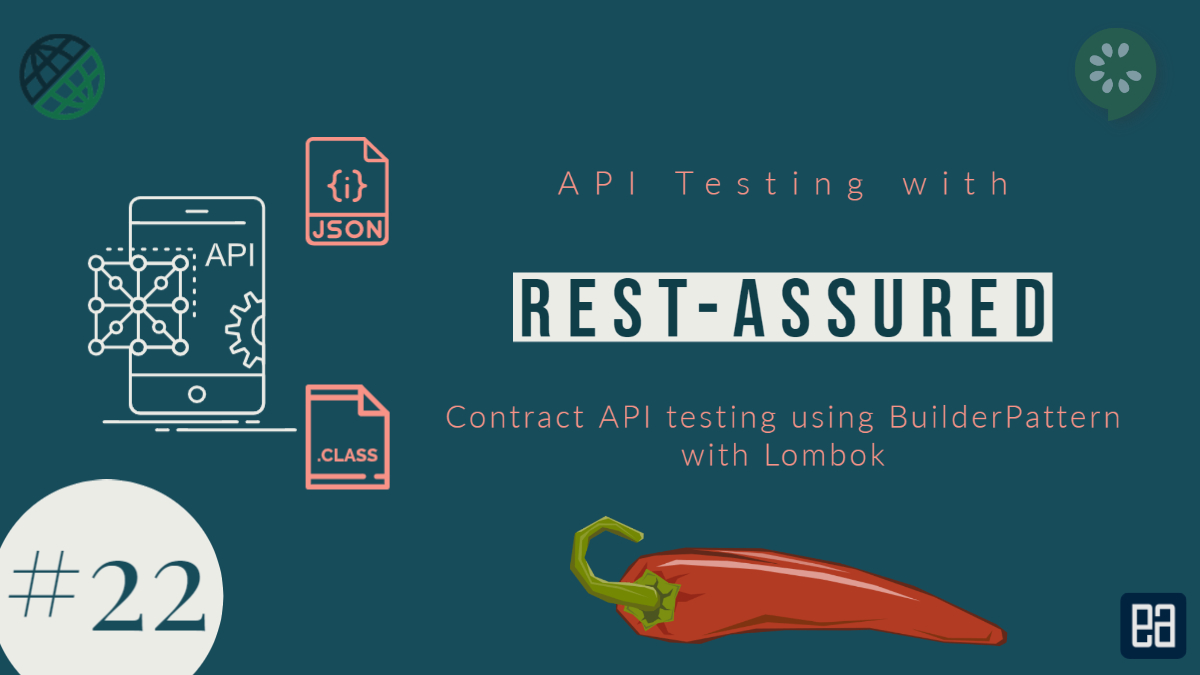
Builder Pattern
The Builder is a design pattern designed to provide a flexible solution to various object creation problems in object-oriented programming. The Builder pattern allows the creation of different representations of an object using the same construction code. The Builder design pattern solves problems like:
- How can a class (the same construction process) create different representations of a complex object?
- How can a class that includes creating a complex object be simplified?
POJO classes without Builder pattern gets very complex due to Telescopic constructors and makes the coding very complex.
Code without Builder pattern
Telescopic constructor hell
The code without builder pattern (the common way) will have Telescopic constructors as shown below
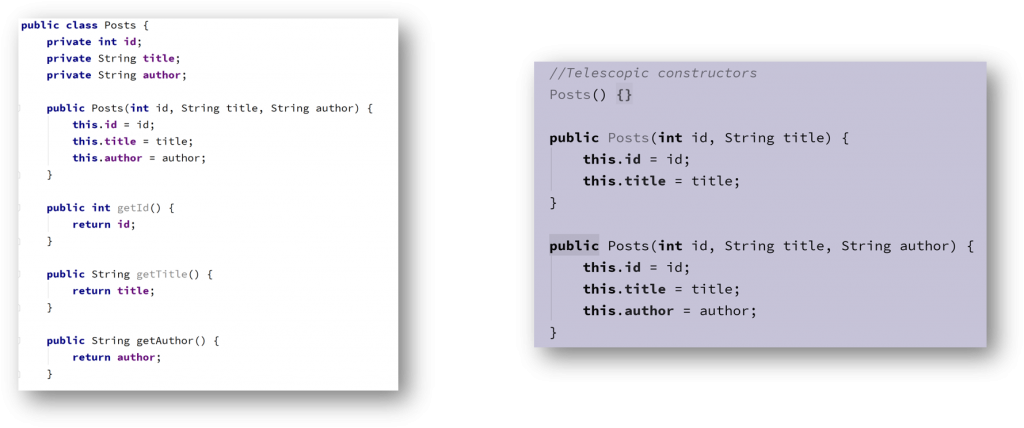
Code with builder pattern
A high level view
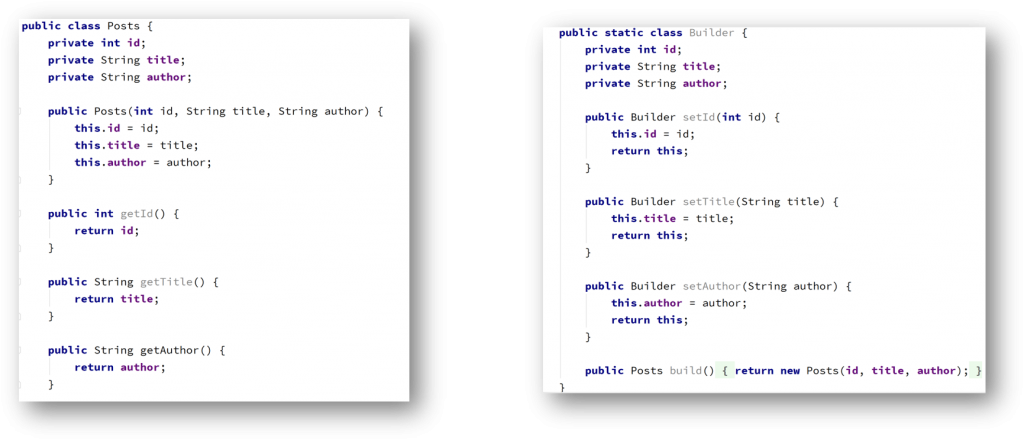
Calling the builder pattern POJO
Calling builder pattern POJO requires one to call the builder method and then access its methods as shown for posts.getAuthor()
var posts = new Posts.Builder().build() var post = response.getBody().as(posts.getClass()); var author = posts.getAuthor()
Code with Lombok plugin
Project Lombok’s @Builder is a useful mechanism for using the Builder pattern without writing boilerplate code. We can apply this annotation to a Class or a method.Lombok plugin
To work with Lombok plugin of builder pattern in IntelliJ, we need to install the plugin in IntelliJ IDE and add an dependency in our project as shown below
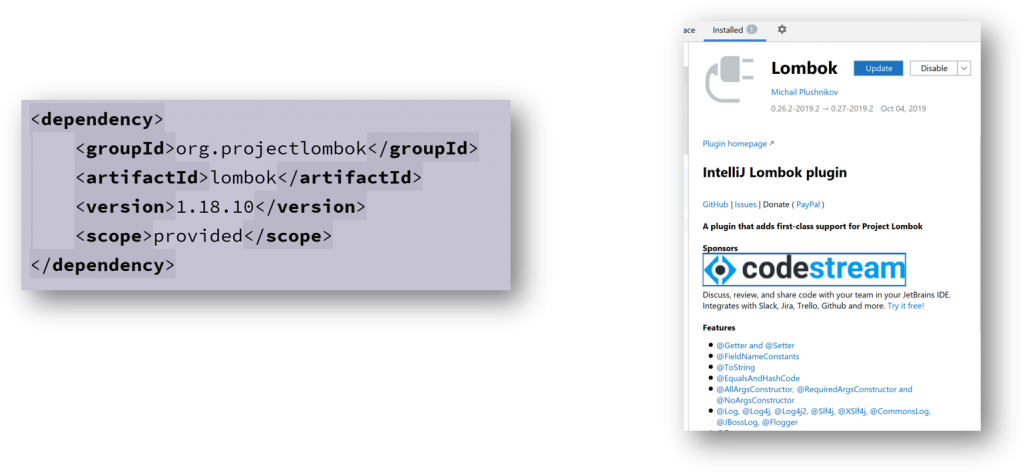
Once the plugin is installed, we can then start using it in our POJO classes. We can replace the above POJO class to something like one shown below
@Data @Builder public class Posts { private int id; private String title; private String author; }
The detailed discussion is available here in this video
Thanks for reading the article and watching those video !
Hope you how contract API testing is done using Builder patterns in real time environments.
Thanks,
Karthik KK
Hi Karthik
Thanks karthik watched the video you had sent on Where do i start Automation.
Indeed a nice compilation.
I wish to start now with the Programming language Java can you send me links to move on.
Do you mean testing in Java like Selenium ?
Hi Karthik,
Thanks for your all videos for Rest Assured its very useful but i am struggling to do POST with authentication is that possible to share with me how to do POST with auth.
Thanks