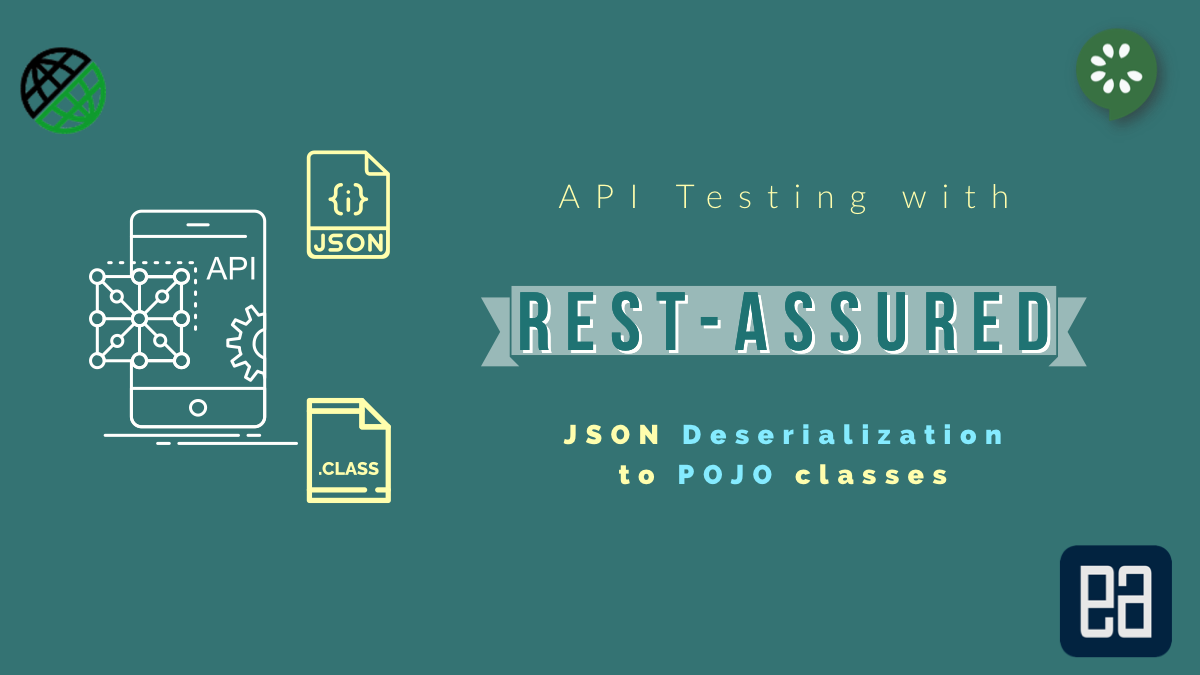
In our last post we discussed how we can deserialize simple JSON response to POJO class, in this post we will discuss how we can deserialize complex JSON response to POJO class
Complex Response
The complex response that we are going to deserialize is this
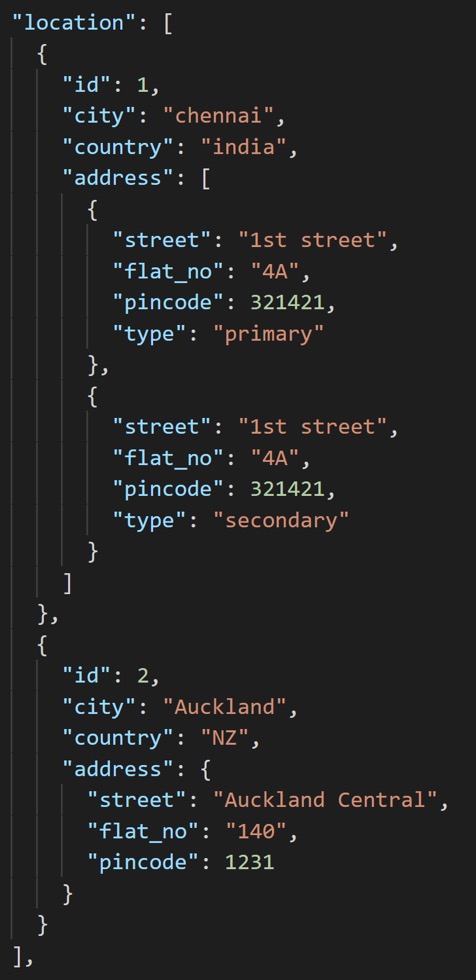
POJO classes
As you can see from the above response it’s very clear to see that it has two nodes
- Location
- Address
Which means, we need to create two POJO classes as shown below, and they need to be an Array as well
package pojo; public class Address { private String street; private String flat_no; private int pincode; private String type; Address() {} public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } public String getFlat_no() { return flat_no; } public void setFlat_no(String flat_no) { this.flat_no = flat_no; } public int getPincode() { return pincode; } public void setPincode(int pincode) { this.pincode = pincode; } public String getType() { return type; } public void setType(String type) { this.type = type; } }
And for Location as
package pojo; import java.util.List; public class Location { private int id; private String city; private String country; private List address; Location() {} public void setId(int id) { this.id = id; } public void setCity(String city) { this.city = city; } public void setCountry(String country) { this.country = country; } public void setAddress(List address) { this.address = address; } public int getId() { return id; } public String getCity() { return city; } public String getCountry() { return country; } public List getAddress() { return address; } }
Here is the complete video of the above discussion
Once again, thank you very much for watching the video and reading the article, if you are interested in the complete video series, please check it out from here