Here is the algorithm
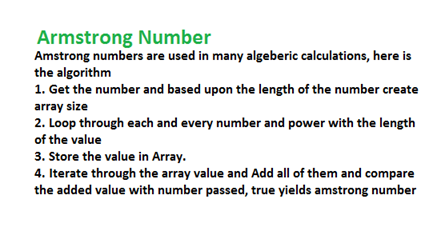
Here is the code snippet
public static bool ArmstrongNumber(int no)
{
//Get the number and its length
string number = no.ToString();
int result = 0;
//Get the length
int len = number.Length;
//Declare the array size
int[] store = new int[number.Length];
for (int i = 0; i < len; i++)
{
//Get the value and parse it to double (used by Math.pow())
double value = double.Parse(number.Substring(i, 1));
double power = double.Parse(len.ToString());
//Power of the Value
store[i] = int.Parse(Math.Pow(value, power).ToString());
}
//Retrive all the value from array
for (int i = 0; i < store.Length; i++)
{
result = result + store[i];
}
//If the added value and actual value are same
if (result == no)
return true;
else
return false;
}
To calculate the Armstrong number from 1 to 999 or 1 to 9999, you can do the following by calling the above method
public static void HowManyArmstrong(int number)
{
for (int i = 0; i <= number; i++)
{
if (ArmstrongNumber(i))
Console.WriteLine("The number " + i + " is Amstrong ");
}
}
Here is the output for value from
0 to 9999
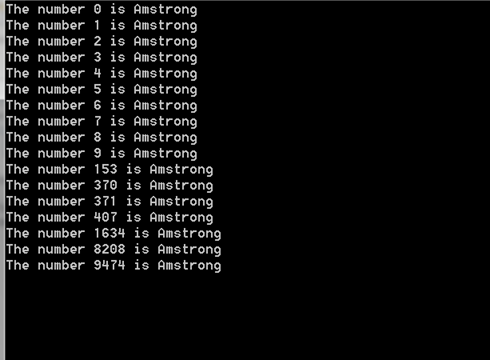
Thanks,
Karthik KK